import java.util.*;
class Char
{
public static void main(String r[])
{
Scanner sc=new Scanner(System.in);
System.out.println("Enter number of characters: ");
int n=sc.nextInt();
String in[]=new String[n];
for(int i=0;i<n;i++)
{
in[i]=sc.next();
}
for(int i= 0;i<n;i++)
{
if(in[i].equals("dle"))
{
in[i]="dle dle";
}
}
System.out.println("Transmitted message is: ");
System.out.print(" dle stx ");
for(int i=0;i<n;i++)
{
System.out.print(in[i]+" ");
}
System.out.println(" dle etx ");
}
}
Example:
Sample output:
Enter number of characters:
5
a b dle dle d e
Transmitted message is:
dle stxa b dle dle dle dle d dle etx
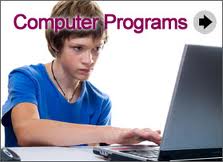
Welcome guys................... This blog is basically created to accumulate and share as much knowledge i can share. I will try to cover as much of topic I can. So just post any program or concept you want to know related to any programming language, Tibco , Algorithm, OOPS, android etc. You are free to post any problem you may face in these programs just post a comment. If you are not able to make any program just post the details and it will be posted to this blog as soon as possible.
Java FAQ
Thursday 11 April 2013
Program to concatinate two strings
Program to concatinate two strings in C
void main()
{
char a[]={"Hello"}; //declare first char array
char b[]={"Everyone"}; //declare second char array
char c[50];
int n,i,j,k=0;
clrscr();
n=sizeof(a)+sizeof(b)-2; //store the total size of both array
for(i=0;i<sizeof(a);i++) //storing first array in the resultant array
c[i]=a[i];
for(i=sizeof(a)-1;i<n;i++,k++) //storing the second array in the resultant array
c[i]=b[k];
for(i=0;i<n;i++)
printf("%c",c[i]); //displat the result
getch();
}
Monday 8 April 2013
New Page added "ASK"
In this page u can ask any question or query or code related to any of the programming language which may be of C,C++,JAVA.
The solution will be posted on the blog as soon as possible...
So don't hesitate and ask anything u want......
visit http://rrprograms.blogspot.in/p/blog-page.html
Thursday 21 March 2013
WAP TO REVERSE A GIVEN SENTENSE
JAVA Programs
import java.util.*;
public class ReverseSentense
{
public static void main(String args[])
{
Scanner sc=new Scanner(System.in);
String s;
System.out.println("Enter a sentense:");
s=sc.nextLine();
String temp[];
temp=s.split(" ");
for(int i=temp.length-1;i>=0;i--)
System.out.print(temp[i]+" ");
}
}
output:-
let the input be:
hello my name is rishabh
then the output will be:
rishabh is name my hello
Sunday 17 March 2013
Program for Bit Destuffing
//Removing a zero(0) after a series of 5 consecutive one's(1)
import java.util.*;
class BitDestuffing
{
public static void main(String args[])
{
Scanner input=new Scanner(System.in);
int []arr=new int[20];
int cnt=0;
int m=0;
System.out.print("Enter string length: ");
int a=input.nextInt();
System.out .print("Enter a string: ");
for(int i=0;i<a;i++)
{
arr[i]=input.nextInt();
}
for(int i=0;i<a;i++)
{
if(arr[i]==1)
cnt++;
else
cnt=0;
if(cnt==5)
{
if(arr[i+1]==0)
i=i++;
m=i+1;
cnt=0;
}
}
System.out.println("Your String is:");
for(int i=0;i<a;i++)
{
if(i==m)
{}
else
System.out.print(arr[i]);
}
}
}
import java.util.*;
class BitDestuffing
{
public static void main(String args[])
{
Scanner input=new Scanner(System.in);
int []arr=new int[20];
int cnt=0;
int m=0;
System.out.print("Enter string length: ");
int a=input.nextInt();
System.out .print("Enter a string: ");
for(int i=0;i<a;i++)
{
arr[i]=input.nextInt();
}
for(int i=0;i<a;i++)
{
if(arr[i]==1)
cnt++;
else
cnt=0;
if(cnt==5)
{
if(arr[i+1]==0)
i=i++;
m=i+1;
cnt=0;
}
}
System.out.println("Your String is:");
for(int i=0;i<a;i++)
{
if(i==m)
{}
else
System.out.print(arr[i]);
}
}
}
Program of BitStuffing
//Inserting a zero(0) after a series of five consecutive one's(1)
import java.util.*;
class BitStuffing
{
public static void main(String args[])
{
Scanner input=new Scanner(System.in);
int []arr=new int[20];
int cnt=0;
System.out.print("Enter string length: ");
int a=input.nextInt();
System.out .print("Enter a string: ");
for(int i=0;i<a;i++)
{
arr[i]=input.nextInt();
}
for(int i=0;i<a;i++)
{
if(arr[i]==1)
cnt++;
else
cnt=0;
if(cnt==5)
{
arr[i]=10;
cnt=0;
}
}
System.out.println("Your String is:");
for(int i=0;i<a;i++)
{
System.out.print(arr[i]);
}
}
}
Wednesday 6 March 2013
Program to find out number of IP's conected to the given website
import java.net.*;
import java.util.*;
class NoOfIp
{
public static void main(String args[])throws Exception
{
Scanner s=new Scanner(System.in);
System.out.println("Enter the url: ");
String site=s.next();
InetAddress[] i=InetAddress.getAllByName(site);
int j;
for(j=0;j<i.length;j++)
System.out.println(i[j]);
System.out.println("Number of ip found are: "+j );
}
}
Program to fetch the source code of a given website
import java.net.*;
import java.util.*;
import java.io.*;
class FetchCode
{
public static void main(String args[])throws Exception
{
Scanner s=new Scanner(System.in);
System.out.println("Enter the url: ");
String site=s.next();
URL u=new URL(site);
BufferedReader br=new BufferedReader(new InputStreamReader(u.openStream()));
String line;
while((line=br.readLine())!=null)
System.out.println(line);
br.close();
}
}
NOTE:- While writting the input URL write its full name for example for FACEBOOK write http://www.facebook.com
Program to obtain the IP address of a given website address.
import java.net.*;
import java.util.*;
class Ip
{
public static void main(String args[])
{
try{
Scanner sc=new Scanner(System.in);
System.out.println("Enter the url");
String url=sc.next();
//String url=args[0]; //By Command line
InetAddress i=InetAddress.getByName(url);
System.out.println("Host address:" +i.getHostAddress());
System.out.println("Host name :"+i.getHostName());
}
catch(Exception e)
{}
}
}
import java.util.*;
class Ip
{
public static void main(String args[])
{
try{
Scanner sc=new Scanner(System.in);
System.out.println("Enter the url");
String url=sc.next();
//String url=args[0]; //By Command line
InetAddress i=InetAddress.getByName(url);
System.out.println("Host address:" +i.getHostAddress());
System.out.println("Host name :"+i.getHostName());
}
catch(Exception e)
{}
}
}
Program to find out you own IP address
import java.net.*;
class MyIp
{
public static void main(String args[])
{
try
{
InetAddress i =InetAddress.getLocalHost();
String name=i.getHostAddress();
String hname=i.getHostName();
System.out.println("Ip address= "+name);
System.out.println("Ip name= "+i);
System.out.println("Host name= "+hname);
}
catch(Exception e)
{}
}
Subscribe to:
Posts (Atom)